ILA¶
The customizable Integrated Logic Analyzer (ILA) IP core is a logic analyzer that can be used to monitor the internal signals of a design. The ILA core includes many advanced features of modern logic analyzers, including boolean trigger equations and edge transition triggers. Because the ILA core is synchronous to the design being monitored, all design clock constraints that are applied to your design are also applied to the components of the ILA core.
Features Selectable at Design Flow Time:
Number of probe ports and port widths
The number of match unit comparators, per port.
Multiple (logical) Probes may use different bit ranges on the same probe port.
Waveform Data sample storage depth.
Trig-In and Trig-Out signal ports.
Features Selectable at Debug Run-Time:
Probe match values.
Global boolean trigger condition.
Waveform window count, window size and Trigger Position.
The ILA class represents one ILA core
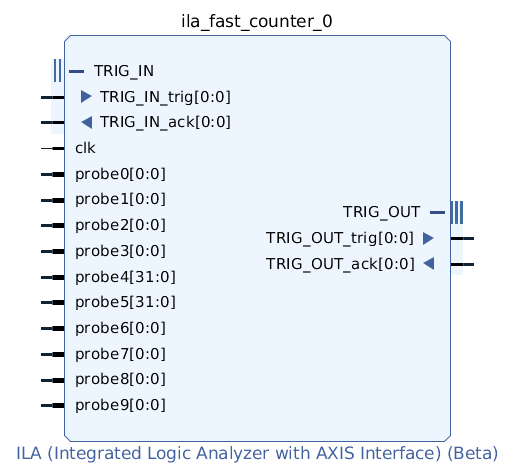
ILA Attributes¶
- class chipscopy.api.ila.ila.ILA(tcf_ila, device, ltx)[source]¶
Data read-only attributes are accessible by dot-notation’, e.g. my_ila.static_info.status
Attribute
Type
Description
name
str
Name of ILA core instance.
core_info
Debug core version information.
static_info
Feature set and dimensions of the ILA core.
status
Dynamic status of ILA capture controller.
control
Trigger setup.
ports
[
ILAPort
]List of ILA core ports.
probes
{str,
ILAProbe
}Probes by probe name.
probe_values
{str,
ILAProbeValues
}Probe compare values.
waveform
Last uploaded waveform data, or None.
ILA Probe Functions¶
There is a logical mapping between ports of an ILA core and elements in the user’s design. This port mapping is recorded in the LTX file in the Vivado design flow.
When an LTX file is read, the HDL net/bus name mapping is available. This enables reading and writing probe values in the context of the HDL design.
ILA.get_probe_capture_value¶
- ILA.get_probe_capture_value(name)[source]¶
Get basic capture mode compare value for a probe.
- Parameters
name (str) – Probe name.
- Return type
[]
- Returns
List with two items [<operator>, <value>]. See
ILAProbeValues
ILA.get_probe_enum¶
ILA.get_probe_trigger_value¶
- ILA.get_probe_trigger_value(name)[source]¶
Get basic trigger mode compare value(s) for a probe.
- Parameters
name (str) – Probe name.
- Return type
[]
- Returns
List of <operator>/<value> pairs. See
ILAProbeValues
ILA.reset_probes¶
- ILA.reset_probes(reset_trigger_values=True, reset_capture_values=True)[source]¶
Reset probe compare values, to dont-cares.
- Parameters
reset_trigger_values (bool) – Resets trigger compare values, for all core probes.
reset_capture_values (bool) – Resets basic capture control compare value, for all probes.
- Return type
None
ILA.set_probe_capture_value¶
- ILA.set_probe_capture_value(name, capture_value)[source]¶
For basic capture mode, set probe compare value to filter samples.
- Parameters
name (str) – Probe name.
capture_value ([]) – List with two items: [<operator>, <value>]. See
ILAProbeValues
- Return type
None
ILA.set_probe_enum¶
- ILA.set_probe_enum(name, enum_def, display_as_enum=True)[source]¶
Set enum values for a probe, by providing a enum class.
- Parameters
name (str) – Probe name.
enum_def (enum.EnumMeta) – Enum class defining enum values, e.g. an enum.IntEnum class.
display_as_enum (bool) – If true, sets probe display index to
ILAProbeRadix.ENUM
- Return type
None
ILA.set_probe_trigger_value¶
- ILA.set_probe_trigger_value(name, trigger_value)[source]¶
For basic trigger mode, set probe compare value(s).
A compare value consists of a pair: <operator> and <value> <value> may be of type int or binary string of the correct bit_width. An empty list is a don’t-care value.
Hex values start with a ‘0x’ prefix. Note! String values for 3-bit probes are always interpreted as binary values, e.g. “0x0”, “0x1”, “0xx”, “011”.
- Example: Test for LSB is ‘0’ on a 4-bit probe.
[‘==’, ‘XXX0’]
- Example: Range check, assuming the global Trigger Condition is ILATriggerCondition.AND:
[‘>=’, ‘0011’, ‘<=’, ‘1010’]
- Example: Range check, assuming the global Trigger Condition is ILATriggerCondition.AND:
[‘>=’, 3, ‘<=’, 10]
- Example: Test equal to any of 3 numbers, assuming the global Trigger Condition is ILATriggerCondition.OR:
[‘==’, ‘0011’, ‘==’, ‘0111’, ‘==’, ‘1111’]
- Example: 4-bit dont-care value.
[‘==’, ‘XXXX’]
- Example: Dont-care value, written as an empty list.
[]
Some probes have associated enum values, which can be used when setting values.
- Example: Use an Enum value.
[‘==’, Colors.BLUE]
- Example: Use Enum name string.
[‘==’, “BLUE”]
- Parameters
name (str) – Probe name.
trigger_value (list) – [<operator>, <value>, …] See
ILAProbeValues
- Return type
None
ILA Run Functions¶
The run functions specifies trigger capture options and arms the ILA core.
ILA.refresh_status¶
ILA.monitor_status¶
- ILA.monitor_status(max_wait_minutes=None, progress=None, done=None)[source]¶
Function monitors ILA capture status and waits until all data has been captured, or until timeout or the function is cancelled. Call this function after arming the ILA, before uploading the waveform. The command operates in synchronous mode if done argument has default value None .
Synchronous Mode
Calling this function in synchronous mode, is the same as calling function ILA.wait_until_done().
Function waits until all data has been captured in the ILA core, or timeout.
Use default argument value None for arguments progress and done .
Returns an ILAStatus object.
Asynchronous Mode
This mode is useful for reporting the capture status to stdout or a GUI.
Function does not block. The main thread continues with the next statement.
Returns a future object, which represents the monitor.
A blocking function should be called later on the future object, in order to wait on the calling thread until the status monitor has completed.
Asynchronous Mode is selected, by specifying a done function, which is called after the function has completed.
If no user defined callback is needed, set done argument to dummy function chipscopy.null_callback , to enable asynchronous mode.
Future Object
When the monitor_status function is called in asynchronous mode, it will return a future object. The future object has blocking attributes and functions, which will block the current thread until the status monitor has completed.
Blocking Attributes:
future.result (None or ILAStatus) - ILAStatus object if capture completed successfully, without timeout.
future.error - (None or Exception) - None if no error otherwise an exception object, e.g. timeout exception.
Non-blocking Attribute:
future.progress (None or ILAStatus) - Access in progress function, to read the ILA capture status.
Blocking Function:
future.wait(timeout=None) - None means wait until status monitor completed. Argument timeout is in seconds.
Non-blocking Function:
future.cancel() - Cancels the status monitor. An exception will be raised, in the thread which called the function ILA.monitor_status .
Progress Function
User-defined function which takes one argument future .
Useful for reporting ILA capture status.
The progress function is only called when the ILA capture status changes.
The progress function is called in the TCF Event Thread. The progress function must not call any ChipScoPy API function, which interacts with the cs_server or the device.
Example:
def monitor_status_done(future): if not future.error: # future.result holds an ILAStatus object. print_status(future.progress)
- Parameters
max_wait_minutes (float) – Max time in minutes for status monitor. If None, status monitor never times out.
progress (progress_fn) – See Asynchronous Mode, above. This function runs in the TCF Event Thread.
done (request.DoneFutureCallback) – Done callback. This function runs in the TCF Event Thread.
Returns (
ILAStatus
or request.CsFutureRequestSync or None):- Return type
ILA.run_trigger_immediately¶
- ILA.run_trigger_immediately(trigger_position=0, window_count=1, window_size=-1, trig_out=<ILATrigOutMode.DISABLED: 0>)[source]¶
Trigger ILA immediately.
- Parameters
trigger_position (int) – sample marker inside the window at which the trigger shall appear. Range [0..window_size-1], default value: 0.
window_count (int) – Number of windows to capture. Default value: 1.
window_size (int) – Number of samples per window. Must be a power-of-two value. Default value:
ILA_WINDOW_SIZE_MAX
trig_out (ILATrigOutMode) – Specify what drives TRIG-OUT port. Default value:
ILATrigOutMode.DISABLED
- Return type
None
ILA.run_basic_trigger¶
- ILA.run_basic_trigger(trigger_position=-1, window_count=1, window_size=-1, trigger_condition=<ILATriggerCondition.AND: 1>, capture_condition=<ILACaptureCondition.ALWAYS: 4>, trig_in=<ILATrigInMode.DISABLED: 0>, trig_out=<ILATrigOutMode.DISABLED: 0>)[source]¶
Trigger using probe compare values.
- Parameters
trigger_position (int) – denotes the middle of the window. Range [-1..window_size-1]. Default value:
ILA_TRIGGER_POSITION_HALF
window_count (int) – Number of windows to capture. Default value: 1.
window_size (int) – Number of samples per window. Must be a power-of-two value. Default value:
ILA_WINDOW_SIZE_MAX
trigger_condition (ILATriggerCondition) – Trigger condition global boolean operator.
capture_condition (ILACaptureCondition) – Capture condition global boolean operator, to filter samples.
trig_in (ILATrigInMode) – Usage of TRIG-IN Port. Default value:
ILATrigInMode.DISABLED
trig_out (ILATrigOutMode) – Specify what drives TRIG-OUT port. Default value:
ILATrigOutMode.DISABLED
- Return type
None
ILA.wait_till_done¶
- ILA.wait_till_done(max_wait_minutes=None)[source]¶
Wait until all data has been captured, or until timeout. Call this function after arming the ILA, before uploading the waveform.
- Parameters
max_wait_minutes (float) – Max time in minutes for command. If None, status monitor never times out.
Returns (
ILAStatus
): ILA capture status.- Return type
ILA Waveform Functions¶
ILA functions to upload waveform from core and export.
ILA.upload¶
export_waveform¶
- ila.export_waveform(export_format='CSV', fh_or_filepath=<_io.TextIOWrapper name='<stdout>' mode='w' encoding='utf-8'>, probe_names=None, start_window_idx=0, window_count=None, start_sample_idx=0, sample_count=None, include_gap=False)¶
Export a waveform in VCD or CSV format, to a file. By default, all samples for all probes are exported, but it is possible to select which probes and window/sample ranges.
- Parameters
waveform (ILAWaveform) – waveform data.
export_format (str) –
Alternatives for output format.
’CSV’ - Comma Separated Value Format. Default.
’VCD’ - Value Change Dump.
fh_or_filepath (TextIOBase, str) – File object handle or filepath string. Default is sys.stdout. If the argument is a file object, closing and opening the file is the responsibility of the caller. If argument is a string, the file will be opened and closed by the function.
probe_names (Optional[List[str]]) – List of probe names. Default ‘None’ means export all probes.
start_window_idx (int) – Starting window index. Default is first window.
window_count (Optional[int]) – Number of windows to export. Default is all windows.
start_sample_idx (int) – Starting sample within window. Default is first sample.
sample_count (Optional[int]) – Number of samples per window. Default is all samples.
include_gap (bool) – Default is False. Include the pseudo “gap” 1-bit probe in the result.
- Return type
None
get_waveform_data¶
- ila.get_waveform_data(probe_names=None, start_window_idx=0, window_count=None, start_sample_idx=0, sample_count=None, include_trigger=False, include_sample_info=False, include_gap=False)¶
Get probe waveform data as a list of int values for each probe. By default all samples for all probes are included in return data, but it is possible to select which probes and window/sample ranges.
- Parameters
waveform (ILAWaveform) – waveform data.
probe_names (Optional[List[str]]) – List of probe names. Default ‘None’ means export all probes.
start_window_idx (int) – Starting window index. Default is first window.
window_count (Optional[int]) – Number of windows to export. Default is all windows.
start_sample_idx (int) – Starting sample within window. Default is first sample.
sample_count (Optional[int]) – Number of samples per window. Default is all samples.
include_trigger (bool) – Include pseudo probe with name ‘__TRIGGER’ in result. Default is False.
include_sample_info (bool) –
Default is False. Include the following pseudo probes in result:
’__SAMPLE_INDEX’ - Sample index
’__WINDOW_INDEX’ - Window index.
’__WINDOW_SAMPLE_INDEX’ - Sample index within window.
include_gap (bool) – Default is False. If True, include the pseudo probe ‘__GAP’ in result. Value 1 for a gap sample. Value 0 for a regular sample.
- Returns (Dict[str, List[int]]):
- Ordered dict, in order:
‘__TRIGGER’, if argument include_trigger is True
‘__SAMPLE_INDEX’, if argument include_sample_info is True
‘__WINDOW_INDEX’, if argument include_sample_info is True
‘__WINDOW_SAMPLE_INDEX’, if argument include_sample_info is True
‘__GAP’, if argument include_gap is True
probe values in order of argument probe_names.
Dict key: probe name. Dict value is list of int values, for a probe.
- Return type
Dict
[str
,List
[int
]]
get_waveform_probe_data¶
- ila.get_waveform_probe_data(probe_name, start_window_idx=0, window_count=None, start_sample_idx=0, sample_count=None)¶
Get waveform data as a list of int values for one probe. By default all samples for the probe are returned, It is possible to select window range and sample range.
- Parameters
waveform (ILAWaveform) – waveform data.
probe_name (str) – probe name.
start_window_idx (int) – Starting window index. Default is first window.
window_count (Optional[int]) – Number of windows to export. Default is all windows.
start_sample_idx (int) – Starting sample within window. Default is first sample.
sample_count (Optional[int]) – Number of samples per window. Default is all samples.
- Returns (List[int]):
List probe values.
- Return type
List
[int
]
ILA Data Defintions¶
Constant to indicate trigger position in the middle of the waveform window.
- ila.ILA_TRIGGER_POSITION_HALF = -1¶
Constant to indicate maximum window size.
- ila.ILA_WINDOW_SIZE_MAX = -1¶
CoreInfo¶
- class chipscopy.api.CoreInfo(core_type, drv_ver, core_major_ver, core_minor_ver, tool_major_ver, tool_minor_ver, uuid)[source]¶
Debug Fabric Core version information.
- core_major_ver: int¶
Core IP major version.
- core_minor_ver: int¶
Core IP minor version.
- core_type: int¶
Core type number.
- drv_ver: int¶
SW/IP interface version.
- tool_major_ver: int¶
Major version of the design tool, which created the core.
- tool_minor_ver: int¶
Minor version of the design tool, which created the core.
- uuid: str¶
Core UUID. UUID is unique among debug cores in the design.
ILABitRange¶
ILACaptureCondition (enum)¶
- class chipscopy.api.ila.ILACaptureCondition(value)[source]¶
For Basic Capture Control, the global boolean operator of participating probe comparators.
Enum Value
Description
ALWAYS
Capture all samples.
AND
AND probe comparators to filter data.
OR
OR probe comparators to filter data.
NAND
NAND probe comparators to filter data.
NOR
NOR probe comparators to filter data.
ILAControl¶
- class chipscopy.api.ila.ILAControl(capture_condition, trig_in_mode, trig_out_mode, trigger_condition, trigger_position, trigger_state_machine, window_count, window_size)[source]¶
Trigger setup
- capture_condition: chipscopy.api.ila.ila_capture.ILACaptureCondition¶
Basic capture cntrol global boolean operator. See
ILACaptureCondition
- trig_in_mode: chipscopy.api.ila.ila_capture.ILATrigInMode¶
Usage of TRIG-IN port. See
ILATrigInMode
- trig_out_mode: chipscopy.api.ila.ila_capture.ILATrigOutMode¶
Usage of TRIG-OUT port. See
ILATrigOutMode
- trigger_condition: chipscopy.api.ila.ila_capture.ILATriggerCondition¶
Global boolean operator, for trigger probes. See
ILATriggerCondition
- trigger_position: int¶
Trigger position index, within data window.
- trigger_state_machine: str¶
Trigger State Machine, as a string.
- window_count: int¶
Number of data windows.
- window_size: int¶
Sample count for a window.
ILAPort¶
- class chipscopy.api.ila.ILAPort(bit_width, data_bit_index, index, is_trigger, is_data, match_unit_count, match_unit_type)[source]¶
Probe Port on the ILA Core
- bit_width: int¶
Number of port bits.
- data_bit_index: int¶
Start bit index in waveform data.
- index: int¶
Port index
- is_data: bool¶
Port is used for capturing data.
- is_trigger: bool¶
Port is used for triggering.
- match_unit_count: int¶
Number of compare match units, available to the port.
- match_unit_type: chipscopy.api.ila.ila_probe.ILAMatchUnitType¶
Type of match unit.
ILAProbe¶
- class chipscopy.api.ila.ILAProbe(name, bit_width, is_data, is_trigger, map, mu_count, is_bus=False, bus_left_index=None, bus_right_index=None)[source]¶
Logical probe. Probes may share the same probe port.
- bit_width: int¶
Number of probe bits.
- bus_left_index: Optional[int] = None¶
Bus left index. E.g. 5 in probe
counter[5:0]
- bus_right_index: Optional[int] = None¶
Bus right index. E.g. 0 in probe
counter[5:0]
- is_bus: bool = False¶
True for bus probes
- is_data: bool¶
Probe is used to capture waveform data.
- is_trigger: bool¶
Probe can be used in trigger settings.
- map: str¶
How probe is mapped to ports.
- mu_count: int¶
Max number of compare match units, available to the probe.
- name: str¶
Name of probe.
ILAProbeValues¶
- ila.ILA_MATCH_OPERATORS = ['==', '!=', '>', '<', '>=', '<=', '||']¶
Operators for probe compare values.
Operator |
Description |
---|---|
== |
Equal |
!= |
Not equal |
< |
Less than |
<= |
Equal or less than |
> |
Greater than |
>= |
Equal or Greater than |
|| |
Reduction OR |
- ila.ILA_MATCH_BIT_VALUES = '01xXrRfFnNbBtTlLsS_'¶
Binary bit values for probe compare values.
Bit Value |
Description |
---|---|
_ |
Underscore separator for readability. |
X |
Don’t care bit matches any bit-value. |
0 |
Zero |
1 |
One |
F |
Falling. Transition 1 -> 0 |
R |
Rising. Transition 0 -> 1 |
L |
Laying. Opposite to R. |
S |
Staying. Opposite to F. |
B |
Either Falling or Rising. |
N |
No change. Opposite to B. |
- class chipscopy.api.ila.ILAProbeValues(bit_width, trigger_value, capture_value, display_radix=<ILAProbeRadix.HEX: 2>, enum_def=None)[source]¶
A compare value consists of a pair: <operator> and <value> <value> may be of type int or binary/hex string of the correct bit_width.
Hex values start with a ‘0x’ prefix. Note! String values for 3-bit probes are always interpreted as binary values, e.g. “0x0”, “0x1”, “0xx”, “011”.
An empty list is a don’t-care value.
- Example: Test for LSB is ‘0’ on a 4-bit probe.
[‘==’, ‘XXX0’]
- Example: Range check, assuming the global Trigger Condition is ILATriggerCondition.AND:
[‘>=’, ‘0x3’, ‘<=’, ‘0x10’]
- Example: Range check, assuming the global Trigger Condition is ILATriggerCondition.AND:
[‘>=’, 3, ‘<=’, 10]
- Example: Test equal to any of 3 numbers, assuming the global Trigger Condition is ILATriggerCondition.OR:
[‘==’, ‘0011’, ‘==’, ‘0111’, ‘==’, ‘1111’]
- Example: 4-bit dont-care value.
[‘==’, ‘XXXX’]
- Example: Dont-care value, written as an empty list.
[]
- bit_width: int¶
Number of probe bits.
- capture_value: ]¶
Basic capture compare value. A two item list with [<operator>, <value>]
- display_radix: chipscopy.api.ila.ila_probe.ILAProbeRadix = 2¶
Display radix, when exporting waveform data. Default is ILAProbeRadix.HEX
- enum_def: Optional[enum.EnumMeta] = None¶
int} enum values, for this probe.
- Type
Enum class defining {name
- trigger_value: ]¶
Trigger compare values, in a list.
ILAStaticInfo¶
- class chipscopy.api.ila.ILAStaticInfo(data_depth, data_width, has_advanced_trigger, has_capture_control, has_trig_in, has_trig_out, match_unit_count, port_count)[source]¶
Feature set and dimensions of the ILA core.
- data_depth: int¶
Data depth in samples.
- data_width: int¶
Waveform data sample width, in bits.
- has_advanced_trigger: bool¶
ILA has advanced trigger mode.
- has_capture_control: bool¶
ILA has basic capture control.
- has_trig_in: bool¶
ILA has TRIG-IN port.
- has_trig_out: bool¶
ILA has TRIG-OUT port.
- match_unit_count: int¶
Total number of probe compare match units.
- port_count: int¶
Number of probe ports.
ILAStatus¶
- class chipscopy.api.ila.ILAStatus(capture_state, is_armed, is_full, is_trigger_at_startup, samples_captured, windows_captured, samples_requested, windows_requested, trigger_position_requested)[source]¶
Dynamic status of ILA capture controller
- capture_state: chipscopy.api.ila.ila_capture.ILAState¶
Capture state. See
ILAState
- is_armed: bool¶
Trigger is armed.
- is_full: bool¶
Data buffer is full.
- is_trigger_at_startup: bool¶
ILA is armed at startup.
- samples_captured: int¶
Number of samples captured in current data window.
- samples_requested: int¶
Number of samples per window, as requested when was ILA armed.
- trigger_position_requested: int¶
Trigger position, as requested when ILA was armed.
- windows_captured: int¶
Number of fully captured data windows.
- windows_requested: int¶
Number of windows, as requested when ILA was armed.
ILAState (enum)¶
ILATriggerCondition (enum)¶
- class chipscopy.api.ila.ILATriggerCondition(value)[source]¶
For Trigger Condition, set trigger mode including basic trigger global boolean operator of participating probe comparators
Enum Value
Description
AND
Basic Trigger mode: AND probe comparators.
OR
Basic Trigger mode: OR probe comparators.
NAND
Basic Trigger mode: NAND probe comparators.
NOR
Basic Trigger mode: NOR probe comparators.
IMMEDIATELY
Trigger now.
TRIGGER_STATE_MACHINE
Advanced Trigger mode: TSM file decides when to trigger.
ILATrigInMode (enum)¶
ILATrigOutMode (enum)¶
- class chipscopy.api.ila.ILATrigOutMode(value)[source]¶
Choices for Trig-Out port.
Enum Value
Description
DISABLED
Disables the TRIG_OUT port.
TRIGGER_ONLY
Result of the basic/advanced trigger condition.
TRIG_IN_ONLY
Propagates the TRIG_IN port to the TRIG_OUT port.
TRIGGER_OR_TRIG_IN
OR-ing of the basic/advanced trigger and TRIG_IN port.
ILAWaveform¶
- class chipscopy.api.ila.ILAWaveform(width, sample_count, trigger_position, window_size, probes, data, gap_index=None)[source]¶
Waveform data, with data probe information.
- data: bytearray¶
Waveform data.
Samples are aligned on byte boundary.
This formula can be used to read a bit from the data:
bytes_per_sample = len(data) // sample_count def get_bit_value(data: bytearray, bytes_per_sample: int, sample_index: int, data_bit_index: int) -> bool: byte_value = data[sample_index * bytes_per_sample + data_bit_index // 8] mask = 1 << (data_bit_index & 0x7) return (byte_value & mask) != 0
- gap_index: Optional[int] = None¶
None or 0, if the waveform has no gaps. If the value is >0, one sample bit is reserved to indicate which samples are gaps, i.e. the samples with unknown values. ‘gap_index’ gives the bit location within the sample data.
- probes: Dict[str, chipscopy.api.ila.ila_waveform.ILAWaveformProbe]¶
Dict of {probe name, waveform probe} See
ILAWaveformProbe
- sample_count: int¶
Number of data samples.
- set_sample(sample_index, sample)[source]¶
Sample may have more bytes than waveform samples have. Erase any gap bit.
- Return type
None
- trigger_position: List[int]¶
Trigger position index, for each data window.
- width: int¶
Sample bit width.
- window_size: int¶
Number of samples in a window.
ILAWaveformProbe¶
- class chipscopy.api.ila.ILAWaveformProbe(name, map, map_range, is_bus, bus_left_index=None, bus_right_index=None, display_radix=<ILAProbeRadix.HEX: 2>, enum_def=None)[source]¶
Probe location in a data sample.
- bus_left_index: Optional[int] = None¶
Bus left index. E.g. 5 in probe
counter[5:0]
- bus_right_index: Optional[int] = None¶
Bus right index. E.g. 0 in probe
counter[5:0]
- display_radix: chipscopy.api.ila.ila_probe.ILAProbeRadix = 2¶
Display radix, when exporting waveform data. Default is ILAProbeRadix.HEX
- enum_def: Optional[enum.EnumMeta] = None¶
int} enum values, for this probe.
- Type
Enum class defining {name
- is_bus: bool¶
True for bus probes
- map: str¶
Location string
- map_range: List[chipscopy.api.ila.ila_probe.ILABitRange]¶
List of bit ranges. See
ILABitRange
- name: str¶
Name of probe.