QEP Linux Driver APIs¶
QEP Network Device Operations
This section documents the callbacks/functions exported by QEP for supporting network device operations of Linux kernel stack.
-
int
qep_module_init
(void)¶ This is the entry point of the NIC driver.
Parameters
void
- no arguments
Description
- Called when the qep kernel module is inserted into the Linux kernel
- Creates the debugfs directory for QEP (/sys/kernel/debug/qep_dev/) in the host file system
- Initializes QDMA
- Registers the qep kernel module as a PCIe driver
Return
Returns 0 on Success or -EINVAL on Failure
-
void __exit
qep_module_exit
(void)¶ This is the exit point of the NIC driver. This function is called when driver is removed from the kernel. The driver is unregistered, libqdma and debugfs are cleaned up.
Parameters
void
- no arguments
-
int
qep_pci_probe
(struct pci_dev * pdev, const struct pci_device_id * pci_dev_id)¶ This is probe function which is called when Linux kernel detects a PCIe device with vendor ID and device ID registered for this driver with the Linux kernel.
Parameters
struct pci_dev * pdev
- Pointer to PCIe device struct
const struct pci_device_id * pci_dev_id
- Pointer to pci_device_id structure containing the PCIe Vendor ID and Device ID
Description
- Called when the device ID and vendor ID of a PCIe device on the PCIe bus matches with the IDs registered with the Linux kernel during module init
- Creates a debugfs directory for the probed device and creates debugfs files for qep, stmn and cmac
- Maps user bar (BAR2) for accessing STM-N functionality implemented in the HW
- Reads MAC address from HW
- Initializes STM-N, QDMA and CMAC components. The default number of queues supported is minimum of number of CPU cores and number of MSI-X vectors supported (32). The number of queues for Tx/Rx can be changed using ethtool
- Thread to monitor CMAC Rx sync is launched to check for Rx sync every 5 seconds. The link monitoring starts after the device state is up
- Registers as Netdevice with Linux kernel.
Return
Returns 0 on Success or error code on Failure
-
void
qep_pci_remove
(struct pci_dev * pdev)¶ This function is called when PCIe device is removed from the PCIe bus.
Parameters
struct pci_dev * pdev
- Pointer to PCIe Device struct
Description
This function cleans up all the initialized components of the driver
-
int
qep_open
(struct net_device * netdev)¶ This function is called for interface ‘UP’ request.
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
Description
- Called when an interface “up” request is triggered via net-tools such as ifconfig or ip-link
- QDMA Rx and Tx queues are setup in streaming mode with bypass.
- Per Queue NAPI for Rx and tasklet for Tx are enabled.
- The QDMA queues are configured and activated.
- CMAC is enabled
- Link monitoring for CMAC Rx sync is activated
- Rx and Tx queues operations start after CMAC Link is established
Return
Returns 0 on Success or error code on Failure
-
int
qep_stop
(struct net_device * netdev)¶ This function is called when there is a interface ‘down’ request.
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
Description
- Called when an interface “down” request is triggered via net-tools such as ifconfig or ip-link
- QDMA Rx and Tx queues are stopped.
- Per Queue NAPI for Rx and tasklet for Tx are disabled.
- CMAC is disabled
- Link monitoring for CMAC Rx sync is deactivated
Return
Returns 0 on Success or error code on Failure
-
int
qep_start_xmit
(struct sk_buff * skb, struct net_device * netdev)¶ This function is called from networking stack to send a packet
Parameters
struct sk_buff * skb
- Pointer to sk_buff conataining data and metadata for transfer
struct net_device * netdev
- Pointer to net_device struct of the device
Description
- Upon receiving packets from networking stack, .ndo_start_xmit callback of net_device_ops is called with sk_buff passed as an argument.
- Queue in which packet needs to be transmitted is identified from skb.
- The physical address of sk_buff data and fragments are mapped to qdma_pkt_data structure and passed to
qdma_queue_packet_write()
API. - Once the packet has been DMAed, interrupt is raised by QDMA. qep_isr_tx_tophalf which is registered as the callback during initialization is called.
- qep_isr_tx_tophalf determines the queue and schedules a tasklet and acknowledges the interrupt.
- tasklet routine frees the sk_buff.
Return
Returns 0 on Success or error code on Failure
-
int
qep_rx_pkt_process
(unsigned long qhndl, unsigned long quld, unsigned int len, unsigned int sgcnt, struct qdma_sw_sg * sgl, void * udd)¶ This function processes Rx DMA request
Parameters
unsigned long qhndl
- Handle to the queue on which data is received
unsigned long quld
- Pointer to QEP private data
unsigned int len
- Length of the packet data
unsigned int sgcnt
- Number of entries in scatter gatherer list
struct qdma_sw_sg * sgl
- Pointer to scatter gatherer list
void * udd
- User logic metadata
Description
- Upon the arrival of the data packets from CMAC, QDMA will raise interrupt based on interrupt vector configuration.
- QDMA API provides support for registering additional top half processing function in qdma_dev_conf->:c:func:fp_descq_isr_top()
through callback and QEP driver-specific data via qdma_dev_conf->isr_top_param. This configuration shall be done as
part of
qdma_device_open()
API which is called from the probe function. qep_isr_rx_tophalf()
determines the queue, acknowledges the interrupts and schedules a NAPI for the queue- Callback registered with NAPI, when executed, fetches the data from QDMA to SKB buffer and hands over the packet to the Linux kernel stack
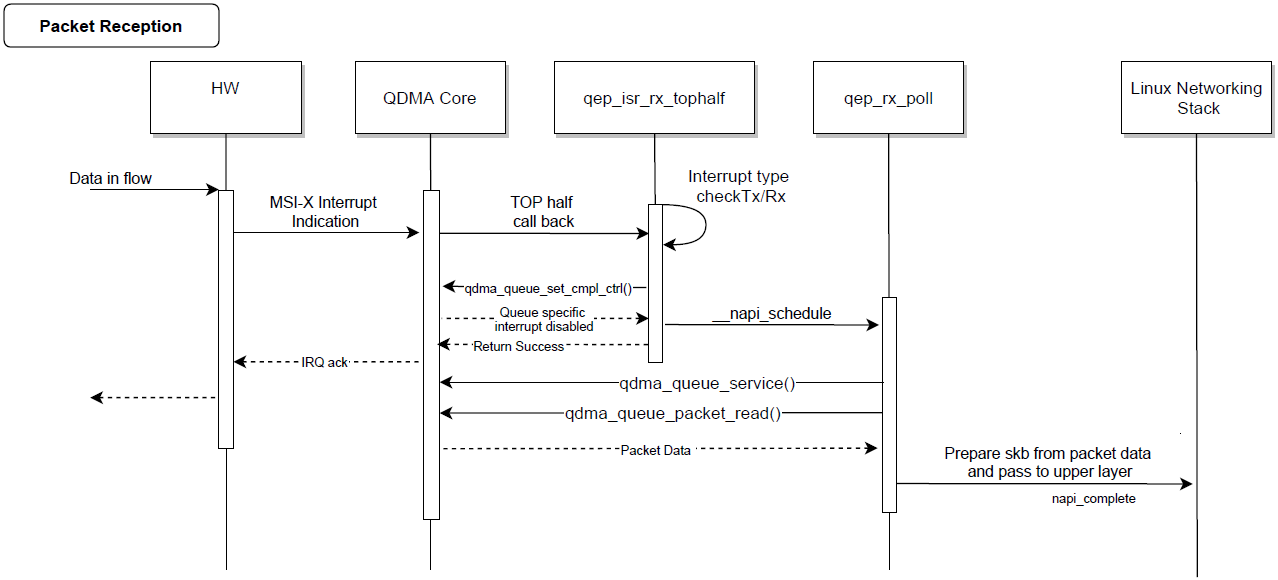
Return
Returns 0 on Success or error code on Failure
-
u16
qep_select_queue
(struct net_device * netdev, struct sk_buff * skb)¶ This function is used to identify the queue from which packet must be transmitted.
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct sk_buff * skb
- Pointer to sk_buff conataining data and metadata for the transfer
Return
queue id on which packet should be queued
-
struct rtnl_link_stats64 *
qep_get_stats64
(struct net_device * netdev, struct rtnl_link_stats64 * stats)¶ This function provides packet statistics.
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct rtnl_link_stats64 * stats
- Pointer to struct rtnl_link_stats64 for link statistics
Return
Returns pointer to rtnl_link_stats64 struct containing stats
-
int
qep_change_mtu
(struct net_device * netdev, int new_mtu)¶ This function changes MTU size of the interface
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
int new_mtu
- New MTU of the device to be set
Return
Returns 0 on Success or error code on Failure
QEP Ethtool Operations
This section documents the callbacks exported by QEP for supporting ethtool operation.
-
void
qep_get_drvinfo
(struct net_device * netdev, struct ethtool_drvinfo * drvinfo)¶ This is an ethtool callback to print driver info
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_drvinfo * drvinfo
- Pointer to struct ethtool_drvinfo
Description
This is to support ethtool option “-i|–driver” which shows driver information. Module name, version and bus info are populated into drvinfo.
-
int
qep_get_regs_len
(struct net_device * netdev)¶ This is an ethtool callback for getting the buffer length required for register dump
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
Return
Buffer length of registers to be dumped.
-
void
qep_get_regs
(struct net_device * netdev, struct ethtool_regs * regs, void * p)¶ This is an ethtool callback for device register dump
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_regs * regs
- Pointer to ethtool_regs struct
void * p
- Pointer to register dump data
Description
This is to support ethtool option “-d|–register-dump” which provides register dump
-
u32
qep_get_msglevel
(struct net_device * netdev)¶ This is an ethtool callback to get the debug message level
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
Return
current dmesg verbosity/level
-
void
qep_set_msglevel
(struct net_device * netdev, u32 data)¶ This is an ethtool callback to set the debug message level
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
u32 data
- Message level
Description
This is to support ethtool option “-s|–change msglvl | msglvl type on|off … ]”
-
void
qep_get_ringparam
(struct net_device * netdev, struct ethtool_ringparam * ring)¶
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_ringparam * ring
- Pointer to ethtool_ringparam struct
Description
This is to support ethtool option “-g|–show-ring” which Query Rx/Tx ring parameters
-
int
qep_set_ringparam
(struct net_device * netdev, struct ethtool_ringparam * ring)¶ This is an ethtool callback to set ring size of Tx and Rx queues
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_ringparam * ring
- Pointer to ethtool_ringparam struct
Description
This is to support ethtool option “-G|–set-ring” which sets Rx/Tx ring parameters
-
void
qep_get_channels
(struct net_device * netdev, struct ethtool_channels * ch)¶ This is an ethtool callback to get number of Rx/Tx queues
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_channels * ch
- Pointer to ethtool_channels struct
Description
This is to support ethtool option “-l|–show-channels” which Query number of Channels.
-
int
qep_get_sset_count
(struct net_device * netdev, int sset)¶ This is an ethtool callback to get count of Tx/Rx stats
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
int sset
- string set ID
Return
number of strings that qep_get_strings will write
-
void
qep_get_strings
(struct net_device * netdev, u32 stringset, u8 * data)¶ This is an ethtool callback to populate set of strings
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
u32 stringset
- string set ID
u8 * data
- Pointer to memory where strings are to be populated, provided by ethtool
Description
This callback only supports ETH_SS_STATS string ID needed to get strings describing the statistics.
-
void
qep_get_ethtool_stats
(struct net_device * netdev, struct ethtool_stats * stats, u64 * data)¶ This is an ethtool callback to get stats
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_stats * stats
- Pointer to ethtool_stats struct
u64 * data
- Pointer to memory where stats are to be populated, provided by ethtool
Description
This is to support ethtool option “-S|–statistics” which shows adapter statistics. Detailed MAC level stats which include good, bad, size based stats, error packets count, etc. is provided.
-
int
qep_get_coalesce
(struct net_device * netdev, struct ethtool_coalesce * ec)¶ This is an ethtool callback to get global coalesce options
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_coalesce * ec
- Pointer to ethtool_coalesce struct
Description
This is to support ethtool option “-c|–show-coalesce” which shows coalesce options.
-
int
qep_set_coalesce
(struct net_device * netdev, struct ethtool_coalesce * ec)¶ This is an ethtool callback to set global coalesce options
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_coalesce * ec
- Pointer to ethtool_coalesce struct
Description
This is to support ethtool option “-C|–coalesce” which sets coalesce options
Return
Returns 0 on Success or error code on Failure
-
int
qep_get_per_queue_coalesce
(struct net_device * netdev, u32 queue, struct ethtool_coalesce * ec)¶ This is an ethtool callback to get per queue coalesce options
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
u32 queue
- queue ID for which configuration is provided
struct ethtool_coalesce * ec
- Pointer to ethtool_coalesce struct
Description
- This is to support ethtool option “-c|–show-coalesce” which shows coalesce options.
- Only rx-usecs(Timer Mode) and rx-frames(counter mode) are valid.
Return
Returns 0 on Success or error code on Failure
-
int
qep_set_per_queue_coalesce
(struct net_device * netdev, u32 queue, struct ethtool_coalesce * ec)¶ This is an ethtool callback to set per queue coalesce options
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
u32 queue
- queue ID for which configuration is provided
struct ethtool_coalesce * ec
- Pointer to ethtool_coalesce struct
Description
- This is to support ethtool option “-C|–coalesce” which sets coalesce options.
- Only rx-usecs(Timer Mode) and rx-frames(counter mode) are configured in this callback.
Return
Returns 0 on Success or error code on Failure
-
int
qep_get_fecparam
(struct net_device * netdev, struct ethtool_fecparam * fec)¶ This is an ethtool callback to get FEC params
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_fecparam * fec
- Pointer to ethtool_fecparam struct
Description
- This is to support ethtool option “–show-fec” which shows FEC settings.
Return
Returns 0 on Success or error code on Failure
-
int
qep_set_fecparam
(struct net_device * netdev, struct ethtool_fecparam * fec)¶ This is an ethtool callback to set FEC params as AUTO, RS or OFF
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_fecparam * fec
- Pointer to ethtool_fecparam struct
Description
- This is to support ethtool option “–set-fec” which sets FEC settings.
- This is to enable or disable RS-FEC encoding in HW.
Return
Returns 0 on Success or error code on Failure
-
int
qep_get_rxfh_key_size
(struct net_device * netdev)¶ This is an ethtool callback to get the size of the Rx Flow Hash key
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
Description
- This is to support ethtool option “–show-rxfh-indir” which sets FEC settings.
Return
Returns 0 on Success or error code on Failure
-
u32
qep_rss_indir_size
(struct net_device * netdev)¶ This is an ethtool callback to get the size of the Rx Flow Hash Indirection table
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
Description
- This is to support ethtool option “–show-rxfh-indir” which sets FEC settings.
Return
Returns 0 on Success or error code on Failure
-
int
qep_get_rxfh
(struct net_device * netdev, u32 * indir, u8 * key, u8 * hfunc)¶ This is an ethtool callback to get the contents of the Rx Flow Hash key and Rx Flow Hash Indirection table
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
u32 * indir
- Pointer to the RSS indirection table
u8 * key
- Pointer to the Rx Flow hash key
u8 * hfunc
- Pointer to the Rx Flow hash function
Description
- This is to support ethtool option “–show-rxfh-indir” which sets FEC settings.
Return
Returns 0 on Success or error code on Failure
-
int
qep_set_rxfh
(struct net_device * netdev, const u32 * indir, const u8 * key, const u8 hfunc)¶ This is an ethtool callback to set the contents of the Rx Flow Hash Indirection table
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
const u32 * indir
- Pointer to the RSS indirection table
const u8 * key
- Pointer to the Rx Flow hash key
const u8 hfunc
- Pointer to the Rx Flow hash function
Description
- This is to support ethtool option “–set-rxfh-indir” which sets FEC settings.
- Setting of the Rx Flow hash key is not supported
Return
Returns 0 on Success or error code on Failure
-
int
qep_get_rxnfc
(struct net_device * netdev, struct ethtool_rxnfc * cmd, u32 * rule_locs)¶ This is an ethtool callback to get Rx flow classification rules
Parameters
struct net_device * netdev
- Pointer to net_device struct of the device
struct ethtool_rxnfc * cmd
- Command to get RX flow classification rules
u32 * rule_locs
- Array of used rule locations
Description
- This is to support ethtool option “–show-nfc” which sets FEC settings.
Return
Returns 0 on Success or error code on Failure